The world of data science is evolving at an unprecedented rate, with organizations across industries recognizing the value of using data to drive decision making. From predicting customer behavior and market trends to identifying patterns and anomalies in large datasets, data science has become a vital tool for businesses, governments, and research institutions. And at the core of this data revolution lies a powerful programming language – Python.
Python has emerged as the leading choice for data scientists and analysts due to its versatility, accessibility, and extensive library of tools and frameworks specifically designed for data analysis and machine learning. In this comprehensive guide, we will explore the reasons behind Python’s dominance in the field of data science and demonstrate how it can be used to master the art of data analysis.
Introduction to Data Science
Before we dive into the power of Python for data science, let’s first understand what data science is all about. Data science is an interdisciplinary field that combines statistics, computer science, and domain knowledge to extract insights and make predictions from data. It involves collecting, organizing, analyzing, and interpreting large volumes of data to uncover hidden patterns, relationships, and trends.
In recent years, the demand for data scientists has skyrocketed, with businesses seeking professionals who can use data to inform strategic decisions and gain a competitive advantage. This has led to a surge in data science courses and certifications, and Python has become an essential skill for anyone looking to enter this in-demand field.
Basics of Python Programming
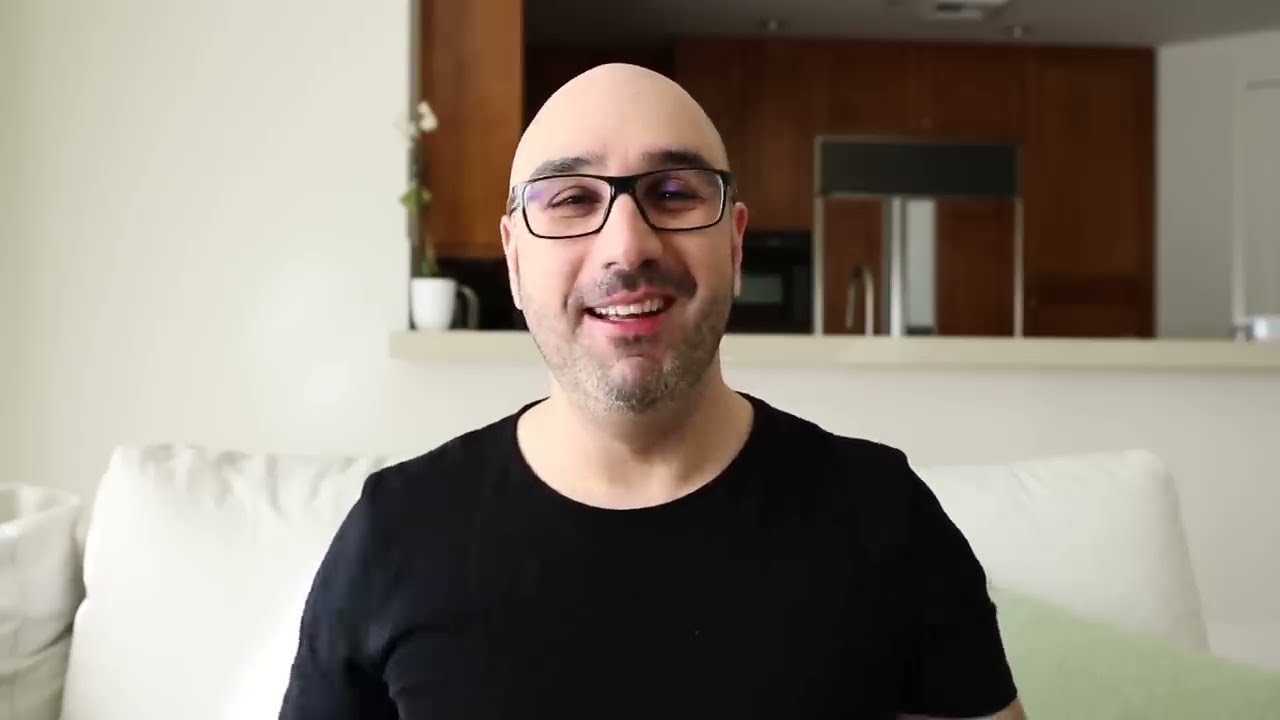
Python is a high-level, general-purpose programming language that was first released in 1991. It has since gained popularity due to its simple syntax, easy-to-learn nature, and vast support community. Unlike other programming languages, Python emphasizes code readability and simplicity, making it accessible even for beginners with no prior programming experience.
Here are some key features of Python that make it an ideal choice for data science:
- Easy to Learn: Python’s simple syntax and clear structure make it easy for beginners to grasp the fundamentals of programming.
- Versatile: Python is a multi-purpose language that can be used for web development, scripting, data analysis, and more.
- Interpreted Language: Unlike compiled languages like Java or C++, Python can be run directly without the need for a separate compilation step.
- Extensive Libraries: Python has a vast library of tools and frameworks that cater specifically to data science tasks, making complex tasks easier and faster to execute.
Understanding Data Science Libraries in Python

Python’s extensive library support is what truly sets it apart as the go-to language for data scientists. These libraries contain pre-written code for specific tasks, allowing users to skip the time-consuming process of writing code from scratch.
Here are some of the essential libraries for data science in Python:
Numpy
Numpy (Numerical Python) is a fundamental package for scientific computing in Python. It provides high-performance multidimensional arrays and tools for working with these arrays. Numpy is an essential tool for data manipulation and forms the basis for many other libraries in Python for data science.
Some of the main features of Numpy include:
- N-dimensional array objects: Numpy offers powerful data structures for working with multi-dimensional datasets, enabling efficient computation and manipulation.
- Broad array of mathematical functions: Numpy has a vast collection of built-in functions for mathematical operations on arrays, such as trigonometric, logarithmic, and exponential functions.
- Broadcasting capabilities: This feature allows for arithmetic operations between arrays of different shapes, making it easier to perform calculations on large datasets.
Pandas
Pandas is a popular library for data manipulation and analysis in Python. It provides high-performance, easy-to-use data structures and tools for handling structured and time-series data. With its intuitive data structures and powerful data manipulation capabilities, Pandas has become an invaluable tool for data scientists.
Some key features of Pandas include:
- Dataframes: Pandas offers the DataFrame data structure, which is a two-dimensional table with labeled columns and rows. It allows for quick and efficient data manipulation and analysis.
- Tools for handling missing data: In real-world datasets, it is common to have missing values. Pandas provides methods for handling these missing values, such as filling them with default values or dropping them altogether.
- Time-series analysis: Pandas has excellent support for manipulating time-series data, making it a go-to library for tasks like forecasting and trend analysis.
Matplotlib
Matplotlib is a comprehensive library for creating charts and graphs in Python. With its intuitive interface and vast customization options, Matplotlib makes it easy for data scientists to create informative and visually appealing visualizations from their data.
Some notable features of Matplotlib are:
- Support for various plot types: Matplotlib offers a wide range of plot types, including line plots, scatter plots, histograms, bar charts, and more.
- Customization options: Users can customize every aspect of their plots, from axes labels and legends to colors and styles, giving them full control over the visualization.
- Seamless integration with other libraries: Matplotlib integrates seamlessly with other data science libraries like Numpy and Pandas, making it easier to visualize data stored in these frameworks.
Data Manipulation and Analysis
Once you have a good understanding of data science libraries in Python, it’s time to put them to use by learning how to manipulate and analyze data using these tools.
Importing Data with Pandas
Pandas provides several functions for importing data from different file formats into a DataFrame. Some commonly used methods are read_csv()
for importing CSV files, read_excel()
for importing Excel files, and read_sql()
for connecting to a SQL database and importing data.
Let’s look at an example of how to import a CSV file using Pandas:
import pandas as pd
# Import the dataset into a DataFrame
df = pd.read_csv('dataset.csv')
# Print the first 5 rows of the dataframe
print(df.head())
Data Cleaning and Preprocessing
Real-world datasets are often messy and contain missing values, irrelevant columns, or duplicate records. Before performing any analysis, it is crucial to clean and preprocess the data to ensure its accuracy and relevance.
Here are some common tasks involved in cleaning and preprocessing data using Pandas:
- Handling Missing Values: As mentioned earlier, Pandas provides methods for filling or dropping missing data. For instance, we can use the
fillna()
function to replace missing values with the mean or median of the column. - Dropping Irrelevant Columns: If a column contains no useful information for our analysis, we can drop it using the
drop()
function. - Removing Duplicates: To remove duplicate records, we can use the
drop_duplicates()
function. - Data Transformation: This involves converting data into a format suitable for analysis, such as changing data types or scaling numerical data.
Grouping and Aggregating Data
Pandas offers powerful functions for grouping and aggregating data. These operations are useful when we want to summarize data based on specific criteria or perform calculations on grouped data.
For example, we can group a DataFrame by a particular column and then perform an aggregation function like sum()
, mean()
, or count()
on the grouped data. Here’s a code snippet that groups data by country and calculates the total population and GDP for each country:
# Group data by country
grouped_df = df.groupby('country')
# Calculate total population and GDP for each country
populations = grouped_df['population'].sum()
gdps = grouped_df['gdp'].sum()
Machine Learning Algorithms in Python
Machine learning is a subset of artificial intelligence that uses algorithms and statistical models to enable systems to learn from data and make predictions or decisions without explicit instructions. Python’s libraries make it easy to implement machine learning algorithms and analyze their performance.
Here are some popular libraries for machine learning in Python:
Scikit-learn
Scikit-learn is a comprehensive library for machine learning in Python. It provides an extensive collection of algorithms and tools for tasks like classification, regression, clustering, and more. With its user-friendly API and detailed documentation, Scikit-learn is an excellent choice for both beginners and experienced data scientists.
Some notable features of Scikit-learn include:
- Easy-to-use API: Scikit-learn offers a consistent API for all its algorithms, making it easier to switch between different models.
- Efficient implementation of algorithms: Scikit-learn’s algorithms are optimized for performance, making it easy to train models even on large datasets.
- Cross-validation and model selection techniques: These functions help choose the best model for a given dataset by evaluating the performance of various models.
TensorFlow
TensorFlow is an open-source library for building and training deep learning models. It provides a flexible platform for creating and deploying machine learning models at scale. TensorFlow is used extensively in industries like healthcare, finance, and manufacturing to develop advanced AI-based products and services.
Some key features of TensorFlow are:
- Comprehensive API: TensorFlow’s Keras API allows users to build complex neural networks with just a few lines of code.
- Distributed Training: TensorFlow supports distributed training, which enables users to train models on multiple machines for better performance.
- TensorBoard: This visualization tool helps users monitor and analyze model performance and identify potential issues.
Data Visualization with Python
Data visualization is the process of creating visual representations of data to communicate insights and conclusions effectively. Visualizations help us understand complex datasets and identify patterns and trends that may not be noticeable in tabular form.
Python offers several libraries for data visualization, but Matplotlib and its interface, Pyplot, are the most commonly used. We have already discussed Matplotlib in the previous section, so let’s now explore another popular library for visualization – Seaborn.
Seaborn
Seaborn is a high-level library built on top of Matplotlib that provides a more appealing and informative interface for creating statistical visualizations. It offers an extensive range of plots and customization options, making it a popular choice among data scientists.
Some key features of Seaborn include:
- Statistical Plots: Seaborn has built-in functions for creating various statistical plots, such as histograms, box plots, and scatter plots.
- Customizable Color Palettes: Seaborn offers a wide range of color palettes to choose from and allows users to create their custom palettes.
- Regression Plots: These plots enable users to visualize the relationship between two variables and fit a regression model to the data.
Advanced Topics in Data Science
So far, we have covered the basics of Python programming and explored some essential libraries and tools for data science. However, there is much more to data science than what we have discussed. Here are some advanced topics you can explore to take your data science skills to the next level:
- Natural Language Processing (NLP): NLP involves using algorithms and techniques to analyze and extract insights from human language in text and speech form. Some popular NLP libraries in Python are NLTK, Spacy, and Gensim.
- Big Data Processing: With the rise of big data, traditional data processing techniques are no longer sufficient. Tools like Apache Spark and Dask offer distributed computing capabilities, making it easier to handle massive datasets.
- Deep Learning: Deep learning is a subset of machine learning that uses artificial neural networks to learn from data. Libraries like TensorFlow and PyTorch provide a powerful platform for developing and deploying deep learning models.
Conclusion and Next Steps
In this comprehensive guide, we have explored the reasons behind Python’s dominance in the field of data science and learned about its essential libraries and tools for data manipulation, analysis, and visualization. We also touched upon advanced topics that you can explore to enhance your skills further.
As with any programming language, mastering data science with Python takes time and practice. But with its user-friendly syntax, extensive library support, and vast community, Python remains an excellent choice for anyone looking to enter the exciting world of data science. So, start exploring, keep learning, and unlock the power of data using Python.